From Lovable To Production: A Developers Guide
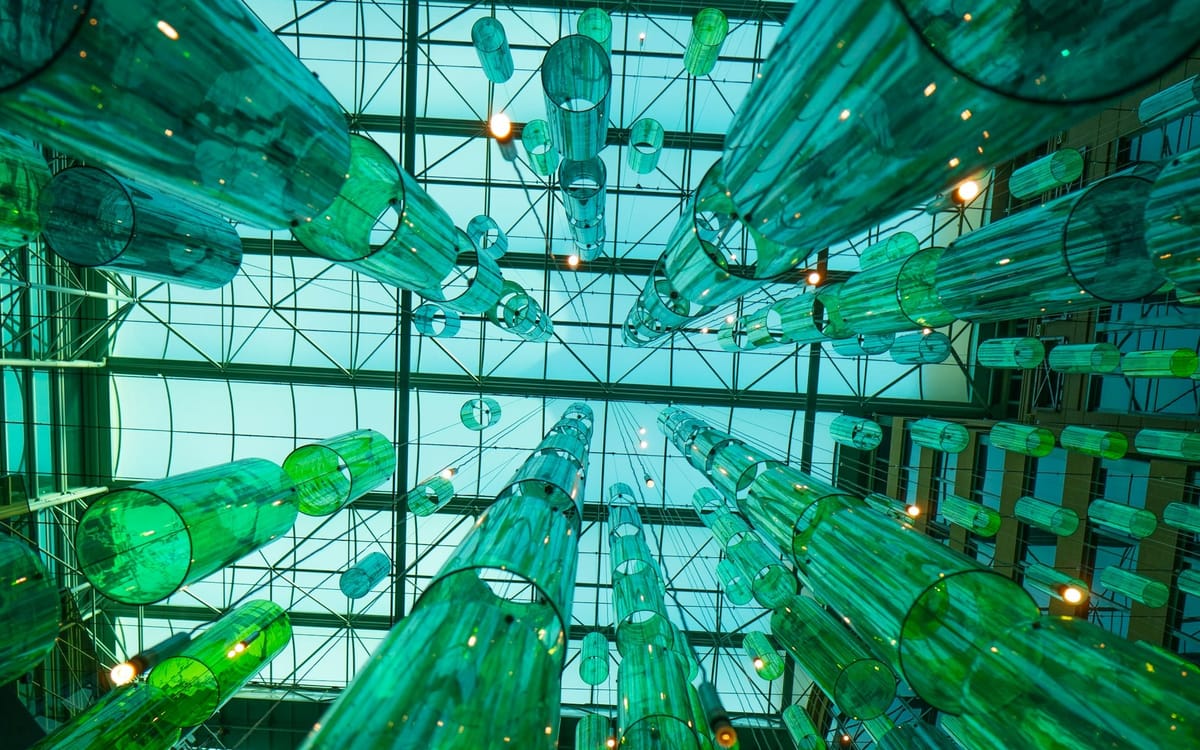
Lovable serves as a fantastic tool for quickly prototyping applications; however, most teams consider it as a temporary solution - build in Lovable first, then completely hand it off to developers to rebuild with traditional coding. But what if you want the collaborative fun to continue?
This guide demonstrates how you can have the best of both worlds by building a Turborepo monorepo architecture around your Lovable application using Git submodules. This powerful approach means:
- Non-technical team members can continue rapidly prototyping and iterating in the Lovable environment they're comfortable with
- Developers can simultaneously build robust production infrastructure around the Lovable app
- Changes sync seamlessly between environments without requiring a complete rebuild
- Your team maintains a collaborative workflow instead of a one-time handoff
The result is a sustainable development process where everyone on your team can contribute effectively using the tools they're most productive with.
Understanding the Workflow
Before diving into the technical steps, let's understand the overall workflow:
- Lovable + GitHub branches for prototyping:
- Your team continues to use Lovable for rapid prototyping
- Changes are made on feature branches, not directly on main
- Pull requests are created to review changes before merging to main
- Turborepo + Submodule for development:
- Your Lovable app exists as a Git submodule inside the Turborepo
- Developers can build around the app (backend, services, packages)
- The main app lives in the original repo, everything else is built around it
- Synchronized development:
- Changes to Lovable can still be made through the Lovable interface
- Technical changes can be made by developers using Cursor or any code editor
- Both workflows coexist without disrupting each other
This approach gives you the freedom to continue rapid prototyping while simultaneously building production-ready infrastructure.
Prerequisites
- A Lovable app ready for handoff
- Basic knowledge of Git and GitHub
- Familiarity with monorepo concepts
- Cursor IDE installed (recommended)
- Node.js and pnpm installed
Step 1: GitHub Integration for Your Lovable App
Since Lovable already syncs with GitHub, focus on setting up a proper branching strategy:
- Understanding Pull Requests:Pull requests are essential for collaborative development. Here's a quick primer:This process is crucial because:
- What is a pull request? It's a way to propose changes from a branch to your main codebase
- Why use them? They enable code review, discussion, and quality control
- After pushing, go to your GitHub repository and you'll see a prompt to create a pull request
- Create the pull request, add a description, request reviewers, and discuss changes
- Once approved, merge the pull request into your main branch
- You don't want to push changes directly to the main branch (causes headaches and overrides)
- A branch-based workflow lets you open your code in Cursor to refine it
- You can review changes before merging them into the main branch
- It establishes good practices for team collaboration
- Ensure your app is at a handoff-ready state with clean code and documentation before proceeding.
Basic workflow:
# Create a new feature branch
git checkout -b feature/new-feature
# Make your changes in Lovable or code editor
# Stage your changes
git add .
# Commit your changes
git commit -m "Add new feature"
# Push your changes to GitHub
git push -u origin feature/new-feature
Set up branches in your Lovable GitHub repository:
# Create a development branch
git checkout -b development
git push -u origin development
Step 2: Setting Up Turborepo
Now that your Lovable app is properly managed in GitHub, it's time to create the production environment:
- Open the project in Cursor IDE for enhanced development capabilities. Cursor significantly simplifies the Devops-style work we're about to do. What might have been finicky and time-consuming becomes straightforward when you know what you're trying to achieve.
- Delete the example apps that come with Turborepo (docs and web are Next.js examples we don't need):
Create a new Turborepo instance:
npx create-turbo@latest your-production-app
cd your-production-app
Clean up the default setup:
rm -rf apps/docs
rm -rf apps/web
Remember, Turborepo gives us a structured monorepo environment that will let us build around our Lovable app while maintaining clean separation of concerns.
Step 3: Integrating Your Lovable App as a Submodule
- What this means: You now have a reference to your Lovable app repository within your Turborepo project. This allows you to:
- Keep your Lovable app code separate but accessible
- Make changes to your Lovable app in its own repository
- Build additional services around your Lovable app
- Important caveat: Code within the submodule cannot directly reference code outside the submodule. This is a limitation of Git submodules.
Add your Lovable app as a Git submodule in the apps directory
git submodule add https://github.com/yourusername/your-lovable-app.git apps/frontend
Managing changes in the submodule:When you need to make changes to your Lovable app from within the Turborepo, you'll follow this workflow:
# Navigate to your Lovable app submodule
cd apps/frontend
# Make changes to files
# Add, commit, and push changes to the Lovable repo
git add .
git commit -m "Update feature X"
git push
# Go back to the root of the Turborepo
cd ../..
# You'll now see that the submodule reference has changed
# Stage, commit, and push this change
git add apps/frontend
git commit -m "Update git submodule commit ID"
git push
This two-step process is crucial for keeping everything in sync. First you update the actual Lovable code, then you update the Turborepo to point to the new version of your Lovable app.
This can be slightly jarring the first few times you do this; but once you get the process down, and write a script that does this, you'll save yourself a ton of time.
Step 4: Building Your Backend Service
Create your server code (example index.js
):
Create a new folder at apps/backend
and set up a new Hono server (or Express, or whatever flavour you enjoy most). It'll look something like this:
Set up a Hono server in this directory:
cd apps/backend
npm init -y
npm install hono @hono/node-server
Do your normal backend server work here:
import { Hono } from 'hono';
import { serve } from '@hono/node-server';
const app = new Hono();
app.get('/', (c) => {
return c.json({ message: 'Hello from your backend!' });
});
// Add your API endpoints here
app.get('/api/content', (c) => {
// Your content generation logic
return c.json({ content: 'Generated content' });
});
const port = process.env.PORT || 3001;
console.log(`Server is running on port ${port}`);
serve({
fetch: app.fetch,
port
});
As standard, you'll want to include rate limiting, IP Safelisting & CORS for specific sites, OpenAPI specs for automatic API generation, and, for a good security measure, middleware that checks for a Supabase Session ID in the header of each request.
The goal is to make sure anyone trying to access your backend have a tougher time doing so, and you'll be less wide open to nefarious behaviour.
Step 5: Connecting Your Lovable App to the Backend
This is a critical step - your Lovable app needs to know how to talk to your backend service both in development and production:
Set up environment variables in your Lovable app:
// apps/frontend/.env.development
VITE_BASE_API_ENDPOINT=http://localhost:3001
// apps/frontend/.env.production
VITE_BASE_API_ENDPOINT=https://your-production-backend-url
Create an API class in your Lovable app:
// apps/frontend/src/api/index.js
export class API {
constructor() {
this.baseUrl = import.meta.env.VITE_BASE_API_ENDPOINT || 'http://localhost:3001';
}
async fetchContent() {
const response = await fetch(`${this.baseUrl}/api/content`);
return response.json();
}
// Add more API methods as needed
}
It's crucial to remember that your Lovable app in production won't have access to localhost! You need to think carefully about your environment variables for different contexts. When deploying, make sure your production API endpoint points to your hosted backend service, not your local development machine.
Step 6: Deployment to Railway
Now it's time to get your application online through Railway.com:
- Sign up and connect to GitHub:
- Create an account on Railway.com
- Connect your GitHub account to Railway
- Grant access to your repositories
- Deploy your backend service:
- From the Railway dashboard, click "New Project"
- Select "Deploy from GitHub repo"
- Choose your Turborepo repository
- Configure the service to build from the
apps/backend
directory - Set up environment variables (PORT, etc.)
- Deploy the service with
pnpm build -F backend
andpnpm start -F backend
, or your Docker file etc.
- Deploy your frontend service:
This is optional but I like having everything served from the same place. If you're into Netlify or Vercel, go for it! The same steps apply.
- Create another GitHub service in the same project
- Select your original GitHub repository (the one from Lovable)
- Set your environment variables (In Railway/Render, use the internal URLs to avoid external costs)
- Deploy the service
- Link your services:
- In the Railway dashboard, you can create a network between your services via Shared Environment Variables
- This allows them to communicate securely
- Update your environment variables to use these internal network addresses if needed
Set the environment variables, including:
VITE_BASE_API_ENDPOINT=https://your-backend-service-url.railway.app
Railway.com is highly recommended for its simplicity and power - it handles both your frontend and backend services smoothly. When properly configured, it will automatically rebuild and deploy your services when you push changes to your GitHub repository.
Alternative deployment options include Vercel, Render, AWS, Netlify, or any other platform of your choice. Remember that regardless of which platform you choose, the key is to properly configure your environment variables and ensure your services can communicate with each other.
Step 7: Advanced Integration Techniques
Sharing Code Between Projects
Since code within the submodule can't directly reference code outside the submodule, you have a few options:
- Lib Directory Method:This approach works better because Lovable will do fresh installs, which would overwrite any changes to node_modules. Using a separate
lib
directory preserves your custom modules.- Create shared packages in your Turborepo
packages
directory - Build these packages with
pnpm build
- Create a
lib
directory in your Lovable app for standalone implementations - Copy the built packages to this
lib
directory instead of node_modules - Import directly from this
lib
directory in your Lovable app
- Create shared packages in your Turborepo
- Use Git Hooks to automate the copy process on commit or push.
Automatic Copy Script:
// scripts/copy-packages.js
const fs = require('fs');
const path = require('path');
const sourceDir = path.resolve(__dirname, '../packages/shared/dist');
const targetDir = path.resolve(__dirname, '../apps/frontend/lib/shared');
// Create target directory if it doesn't exist
fs.mkdirSync(targetDir, { recursive: true });
// Copy files
fs.readdirSync(sourceDir).forEach(file => {
fs.copyFileSync(
path.join(sourceDir, file),
path.join(targetDir, file)
);
});
console.log('Packages copied successfully to lib directory');
Conclusion
Transitioning from a Lovable app to a production-ready environment using Turborepo gives you the best of both worlds: the rapid prototyping capabilities of Lovable and the robust development infrastructure of a monorepo setup.
By following this guide, you can create a seamless development workflow that allows you to continue making changes to your Lovable app while building more complex backend services and shared libraries around it.
Remember that this approach is flexible - as you discover better ways to integrate your services, you can adapt your workflow to meet your specific needs.
Need Help?
If you need assistance getting your Lovable app production-ready, or if you have an app that you want to prepare for launch, get in touch:
Contact: doug@withseismic.com
I've helped clients transition their impressive content generation apps from Lovable prototypes to fully production-ready applications with secure backend servers. Let's get your app over the finish line!